How to calculate percentage increase between two numbers in Excel
In Excel, the calculation of percentage increase holds great value for analyzing financial performance, sales, growth rates, and trends. Furthermore, it proves to be a useful tool for determining percentage-based improvements, such as the enhancement in student marks or any other percentage-related increments across various fields.
The dataset demonstrates the academic performance of the students across two semesters. It showcases varying degrees of improvement, with some students showing significant progress while others have more modest improvements. We will use this dataset to learn how to calculate the percentage increase between two numbers.
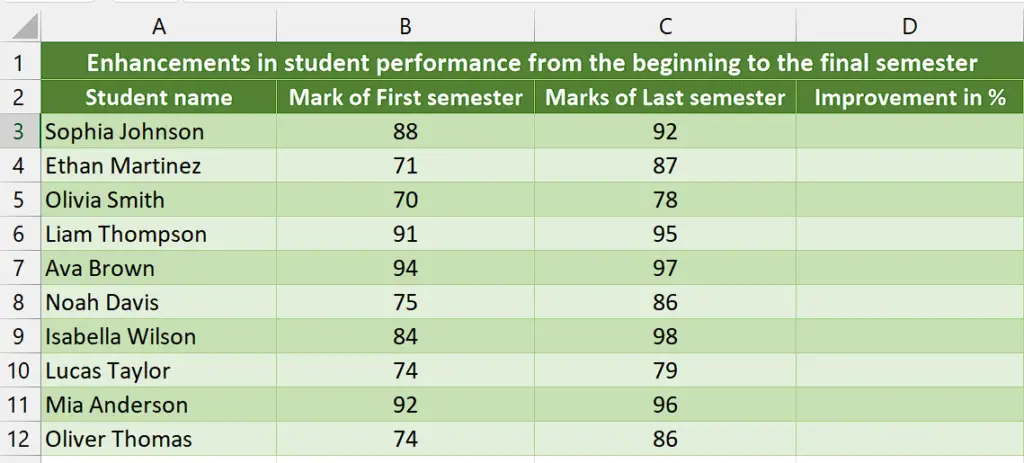
Method 1 – By using simple arithmetic formula
Step 1 – Select the cell
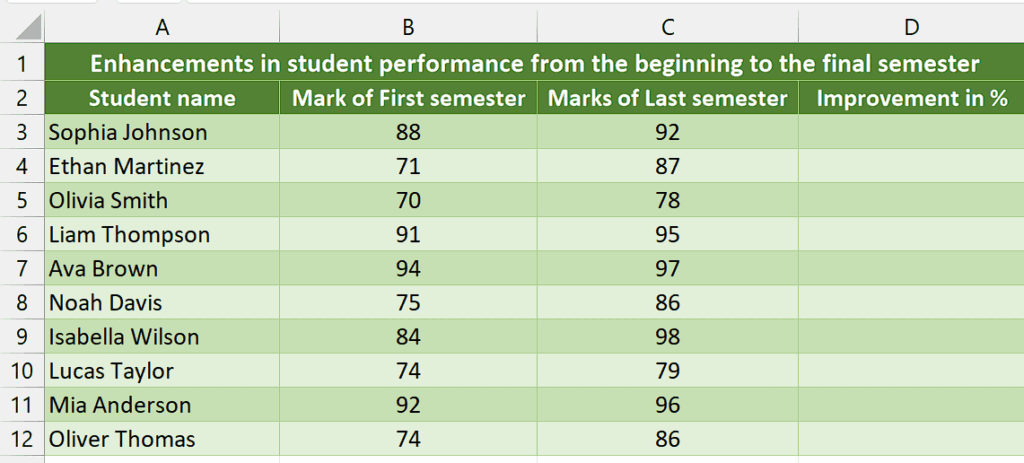
- Select the cell where you wish to calculate the increment in percentage.
Step 2 – Write and implement the formula
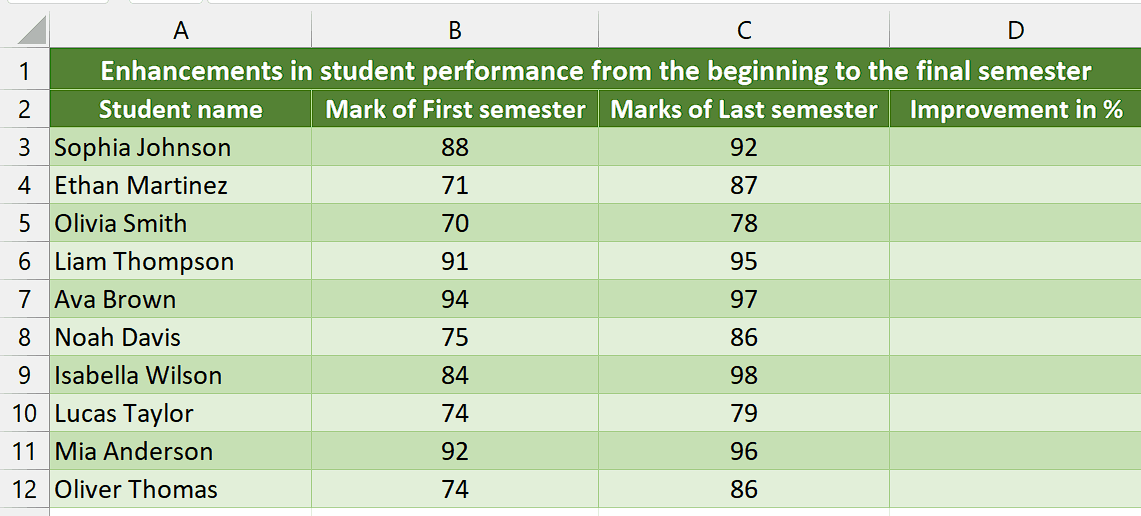
- Write the following formula in the selected cell
=(C3-B3)/B3
Here is the breakdown of the formula,
(C3-B3): This represents the subtraction of the value in cell B3 from the value in cell C3. It calculates the difference between the two numbers.
/B3: This division operation is performed by dividing the result obtained in the above step by the value in cell B3. It normalizes the difference by dividing it by the original value.
- Then, press Enter and the result would appear in the cell.
Step 3 – Format as Percentage
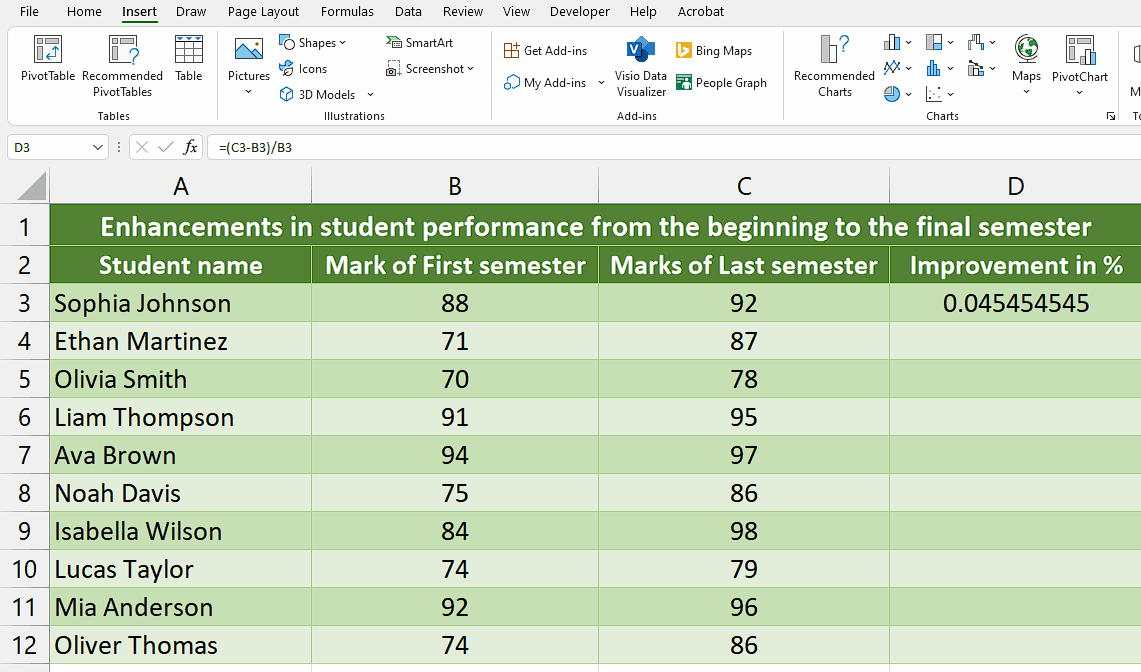
- Select the cell containing the result and navigate to the “Home Tab”.
- Click on the percentage sign (%) in the “Number” tab.
- Now, the cell will be formatted as a percentage.
Step 4 – Implement the formula to the whole range
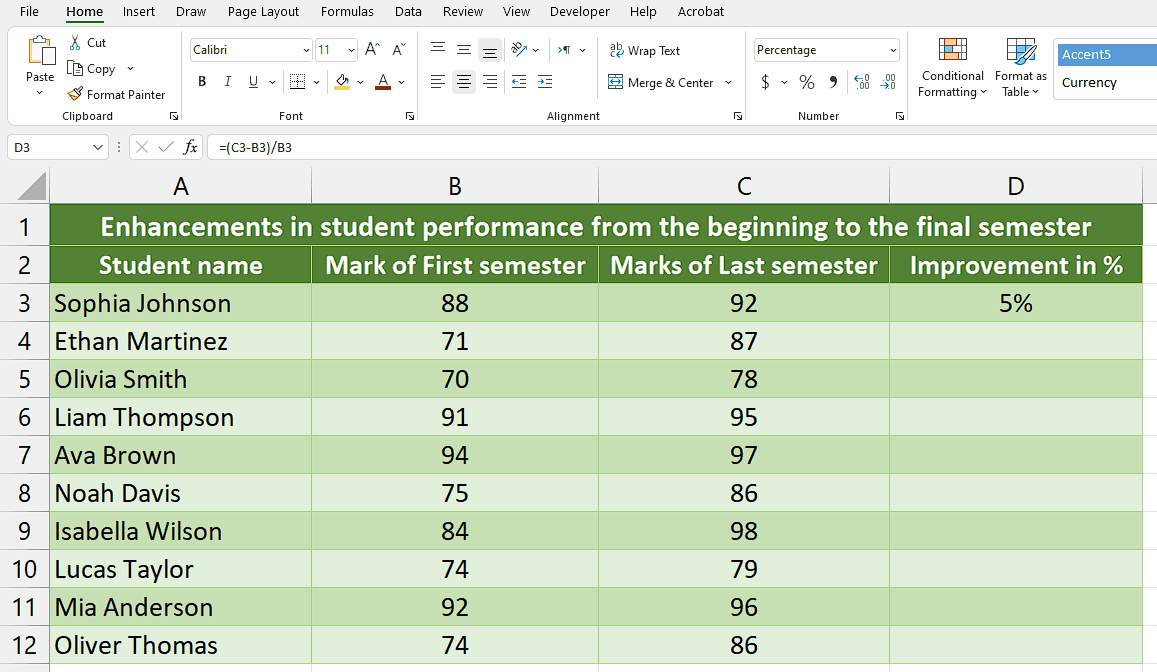
- To apply the formula across a range of cells, begin by selecting the cell containing the desired result, such as cell D3.
- Next, navigate to the bottom right corner of the cell until your cursor transforms into a plus (+) shape, known as the fill handle.
- Double-Click on this fill handle and the formula would be applied to the whole range.
Method 2 – By using the VBA code
Using VBA code to create a user-defined function for calculating the percentage increase between two numbers offers the advantage of automatic formatting of the result as a percentage and providing precise decimal point accuracy.
Step 1 – Insert a module
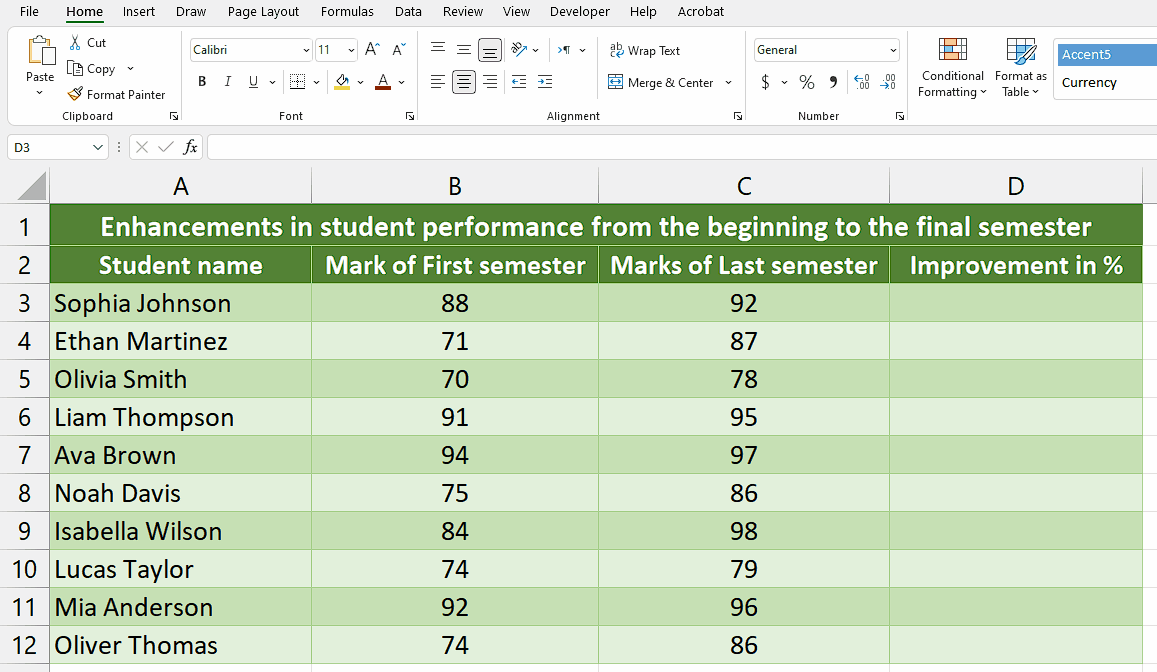
- For adding a module, navigate to the “Developer” tab.
- After that, click on the first option named Visual Basic.
- You’ll see a new window on your screen and you can also open this window by using the shortcut key (Alt+F11) as well.
- Then, click on the Insert tab in this window and click on the Module option.
- Now, a new module would open.
Step 2 – Write the code
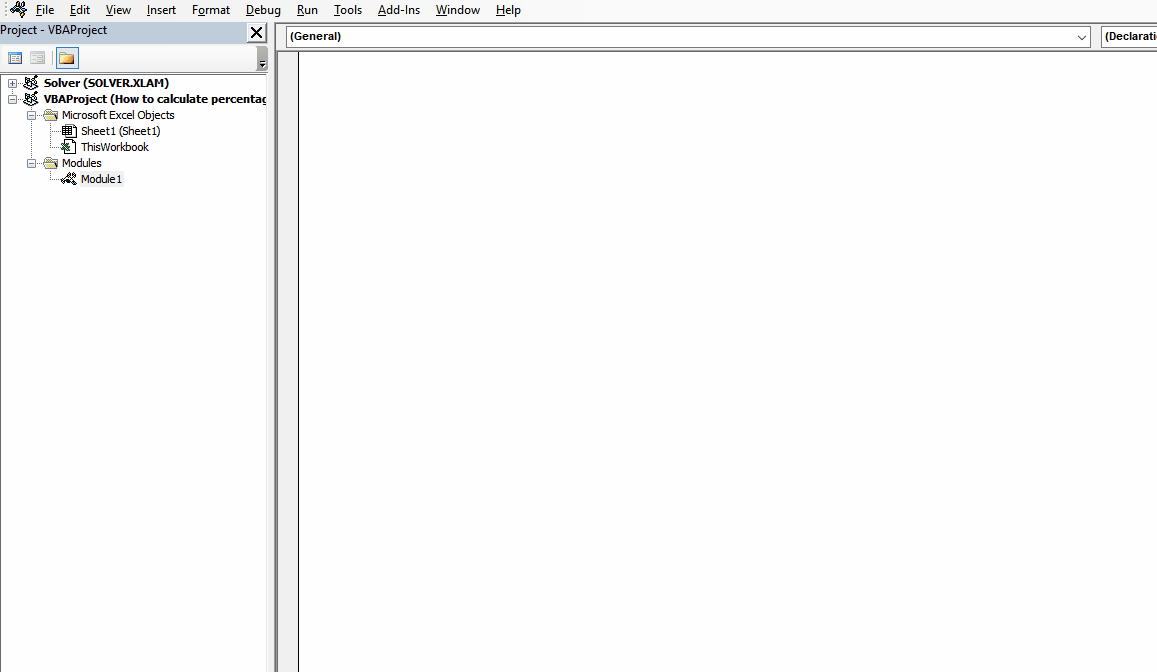
- Write the following code in the module
Function Calc_Percent_Increment(firstNum As Double, secondNum As Double) As String Dim percentageIncrement As Double ‘ Calculate the percentage increment If secondNum <> 0 Then percentageIncrement = ((secondNum – firstNum) / firstNum) Else ‘ Handle the case when the second number is zero percentageIncrement = 0 End If ‘ Format the result as a percentage with two decimal places Calc_Percent_Increment = Format(percentageIncrement, “0.00%”) End Function |
- Then, close the VBA Editor.
Step 3 – Select the cell
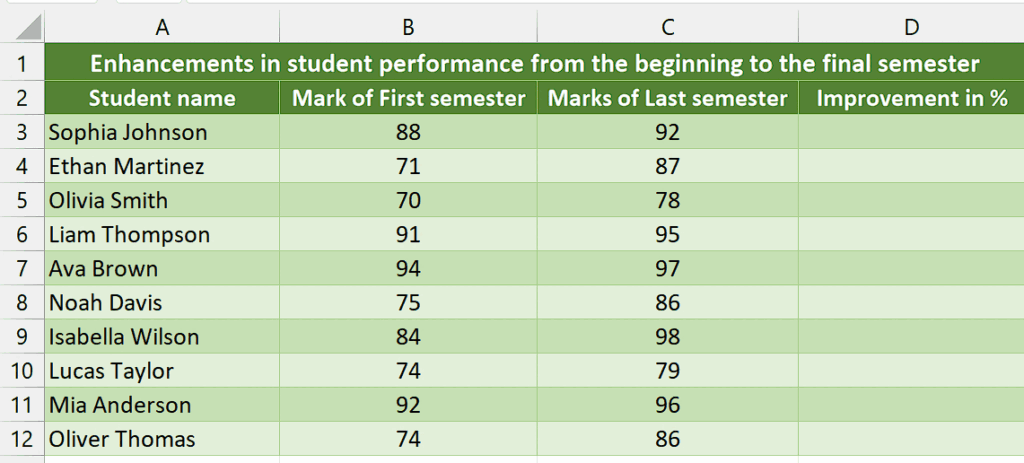
- Select the specific cell where you would like to calculate the percentage increment.
Step 4 – Write and implement the formula

- Now, press the = button on your keyboard.
- After doing that, type “Calc_Percent_Increment” and select the “Calc_Percent_Increment” Function by pressing the tab button.
- Now, input the cell addresses of the first and second numbers.
- Then, your formula would look like this,
=Calc_Percent_Increment(B3,C3)
- Once you’ve followed all the aforementioned steps, press Enter and you’ll get the result in percentage format.
Step 5 – Implement the formula to the whole range
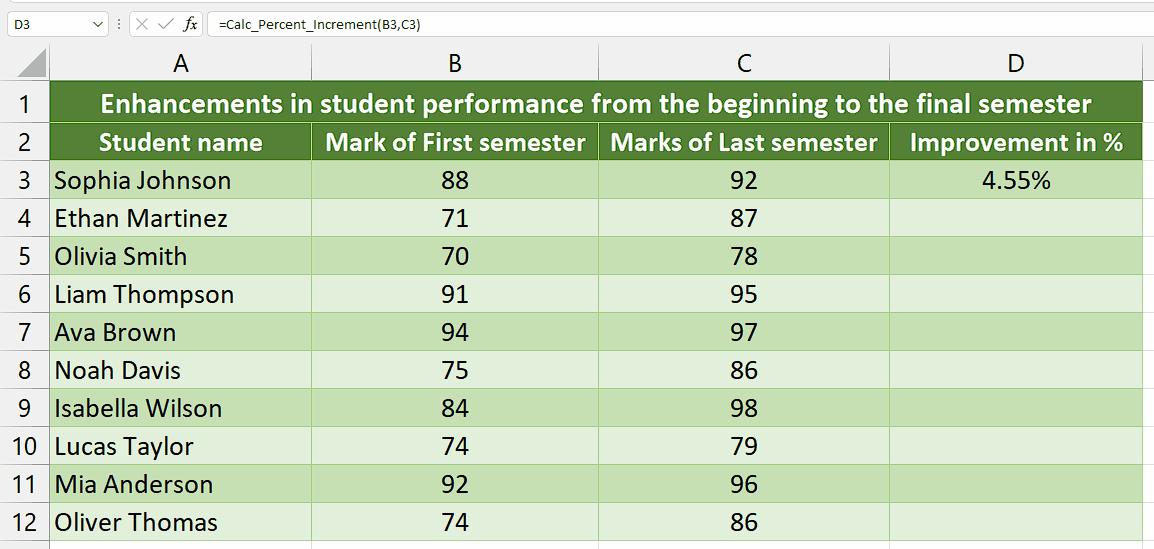
- To apply the formula across a range of cells, begin by selecting the cell containing the desired result, such as cell D3.
- Next, navigate to the bottom right corner of the cell until your cursor transforms into a plus (+) shape, known as the fill handle.
- Double-Click on this fill handle and the formula would be applied to the whole range.
Explanation of code used in Method 2:
The code defines a function called “Calc_Percent_Increment” that takes two numbers, “firstNum” and “secondNum” as parameters. Inside the function, it calculates the percentage increment by subtracting “firstNum” from “secondNum” and dividing the result by “firstNum”. The calculated percentage increment is stored in the “percentageIncrement” variable. If “secondNum” is zero, the code sets “percentageIncrement” to zero to avoid a division by zero error. The code then formats the “percentageIncrement” as a percentage with two decimal places using the Format function. Finally, the calculated and formatted percentage increment is returned as the result of the function.